One of must-have features needed in a Linux kernel (or other system SW) development lab is the ability remotely power cycle lab machines. During bring-up of new kernels or while running tests, if the system hangs, test automation need to time-out the test and power cycle the system to programmatically to move to the next stage. For example, Lava (Linaro Automated Validation Architecture) requires remote PDU capabilities in device under test (DUT). In this article, I describe my implementation of home brewed remote PDU in my home lab using Smart Life devices and publish a simple tool I wrote in python to control these PDUs from anywhere on the internet. The tool works reliably and the latency is often as good as 1 second.
In a previous article, I had described the Wipro Smart Life power plugs I am using with various lab machines in my home lab rack. While the Smart Life mobile app allow power control of these devices, it was necessary to control these devices from a command line tool in order for lab and test automation to work. In order to remotely access all the Smart Life PDU devices in my home lab, I am using Tuya cloud. At a high level, these are the steps I took to facilitate access to the PDU devices using Tuya cloud and program them using a python tool I wrote:
- Create a developer account in Tuya cloud
- Link the Smart Life devices in the home lab from the Smart Life mobile app
- Use the TinyTuya module to access the devices and control them from the python program (powerctl.py)
To use the tool, look at my remote_pdu repository in github. It has the basic documentation on setup and running of the tool.
Before downloading and using powerctl.py, the following steps need to be taken :
Install tinytuya
python -m pip install tinytuya
See TUYA Account section of https://pypi.org/project/tinytuya/ for the next steps for setting up the Tuya developer account. The steps are :
- Create a Tuya account using these instructions – https://github.com/jasonacox/tinytuya/files/12836816/Tuya.IoT.API.Setup.v2.pdf
- Create a Tuya Developer account on https://iot.tuya.com. When it asks for the “Account Type”, select “Skip this step…”
- Create a Tuya cloud project as described in the same section of the guide. You need to choose your region here.
- Click on “Cloud” icon -> Select your project -> Devices -> Link Tuya App Account to link your Smart Life devices.
- Click on Add App Account and it will pop up a Link Tuya App Account dialog to link the Smart Life devices. Choose “Automatic” and “Read only status”, it will still allow commands. It then displays a QR code which needs to be scanned from the Smart Life mobile app (from the Me tab in the app). This can be repeated to add all the Smart Life devices in the Tuya App Account.
- Continue with the steps given in the guide to set up the Service API. IoT Core and Authorization must be added to make this work.
At this point in time, you should be able to view the details of your devices in the Tuya development project you created :
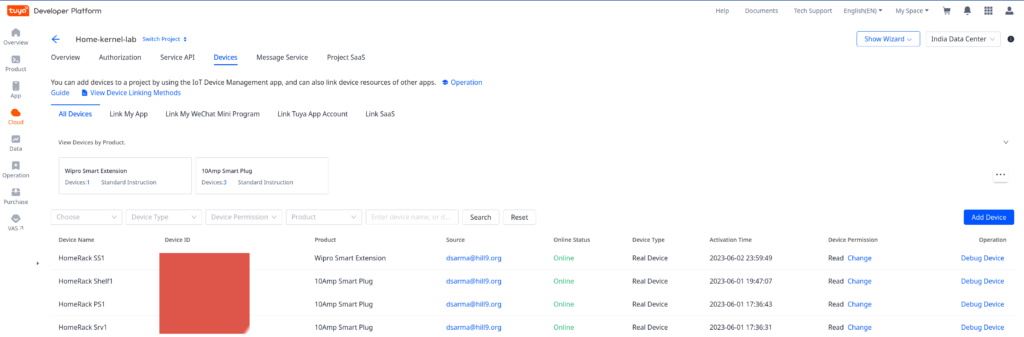
The next step is to use the tinytuya wizard to scan the devices and set up a json database of the devices (devices.json) for ease of lookup and control :
dipankar@conan:~$ python -m tinytuya wizard
TinyTuya Setup Wizard [1.13.2]
Enter API Key from tuya.com: XXXXXXXXXXXX
Enter API Secret from tuya.com: XXXXXXXXXXXX
Enter any Device ID currently registered in Tuya App (used to pull full list) or 'scan' to scan for one: XXXXXXXXXXXX
Region List
cn China Data Center
us US - Western America Data Center
us-e US - Eastern America Data Center
eu Central Europe Data Center
eu-w Western Europe Data Center
in India Data Center
Enter Your Region (Options: cn, us, us-e, eu, eu-w, or in): in
>> Configuration Data Saved to tinytuya.json
{
"apiKey": "XXXXXXXXXXXX",
"apiSecret": "XXXXXXXXXXXX",
"apiRegion": "in",
"apiDeviceID": "XXXXXXXXXXXX"
}
Download DP Name mappings? (Y/n): Y
Device Listing
[
{
"name": "HomeRack PS1",
"id": "XXXXXXXXXXXX",
"key": "XXXXXXXXXXXX",
"mac": "XXXXXXXXXXXX",
"uuid": "XXXXXXXXXXXX",
"sn": "XXXXXXXXXXXX",
"category": "cz",
"product_name": "10Amp Smart Plug",
"product_id": "jwv5jtbscfluha0g",
"biz_type": 18,
"model": "DSP1100",
"sub": false,
"icon": "https://images.tuyain.com/smart/icon/ay1545304748499XogPc/25a35a2b8a1668f67124311d5b50f141.png",
"mapping": {
"1": {
"code": "switch_1",
"type": "Boolean",
"values": {}
},
"9": {
"code": "countdown_1",
"type": "Integer",
"values": {
"unit": "s",
"min": 0,
"max": 86400,
"scale": 0,
"step": 1
}
},
...........
...........
>> Saving list to devices.json
4 registered devices saved
>> Saving raw TuyaPlatform response to tuya-raw.json
Poll local devices? (Y/n): n
Done.
The wizard prompts for Tuya cloud credentials. You can find the API Key (Access ID) and API Secret (Access Secret) from the Authorization tab in your Tuya developer project.
Once the devices.json database is created, clone the remote_pdu repository and use the powerctl.py tool to control the devices.
powerctl.py expects the device data (output of tinytuya wizard) to be stored in a json and also expects the credentials of the cloud project to be stored in a file named cred.json in the following format :
{
"apiKey": "XXXXXXXXXX",
"apiSecret": "XXXXXXXXXXXX",
"apiRegion": "in",
"apiDeviceID": "XXXXXXXXXXXX"
}
The syntax of the tool is as follows :
python powerctl.py [-h] -j JSON_FILE -d DEVICE_NAME -w SWITCH_NAME -c CDOWN_NAME [-t {on,off}] [-s]
DEVICE_NAME is the name of the Smart Life device typically given while setting it up using the mobile app. SWITCH_NAME is the name of a switch that need to be controlled or checked (e.g. switch_1, switch_2 etc). CDOWN_NAME is the countdown field corresponding to the switch to be controlled (e.g. countdown_1, countdown_2 etc.). -t indicates powering on or powering off. If -s is specified, it prints the current status of the switch (On | Off). -t and -s are mutually exclusive. See your devices.json file generated after running the tinytuya wizard.
Some example use of the tool are :
dipankar@conan:~/src/tuya$ python powerctl.py -j devices.json -d "HomeRack SS1" -w switch_2 -c countdown_2 -s
Off
dipankar@conan:~/src/tuya$ python powerctl.py -j devices.json -d "HomeRack SS1" -w switch_2 -c countdown_2 -t on
Sending command...
Results
: {'result': True, 'success': True, 't': 1714111812230, 'tid': 'a4321958039311ef91503a9eb4971f62'}
Switch switch_2 turned on successfully.